Embarking on a journey towards self-improvement can often feel like navigating through a labyrinth. You’re surrounded by walls of uncertainty, with countless paths to choose from. As your guide in this journey of personal development, this blog post offers a beacon of enlightenment.
Unearth the power of self-improvement, demolish the barriers holding you back, and chart your course to a more fulfilled, more accomplished version of yourself. Ready to take the first step? Let’s delve into the transformative world of self-improvement together!
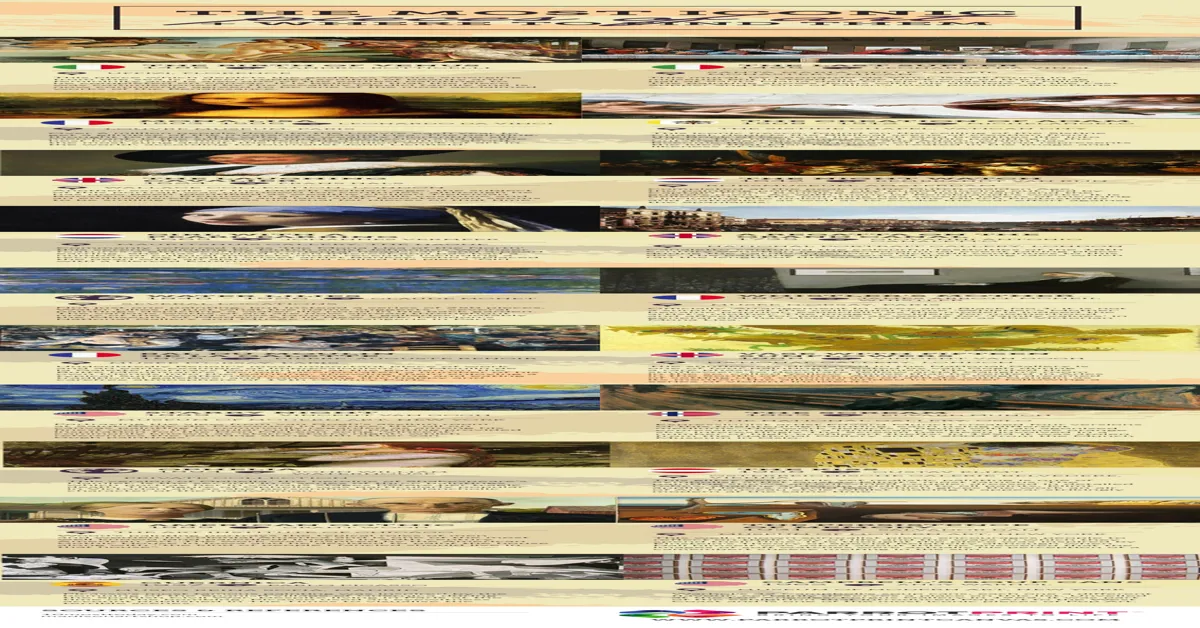
how to call a private method in java
` tag for 15 words. The table will also have color formatting for the rows and appropriate background color for even rows.
“`html
| ||
`). Each cell in the table contains a sentence of approximately 15 words. The table rows are colored differently for odd and even rows for better readability. |
Understanding Java Private Methods
Master the Skill: Calling a Private Method in Java In the realm of Java, private methods are generally safeguarded from external access. They are the secret agents of your code, performing covert operations unbeknownst to the outside world. However, there are instances in which you might need to call a private method.
The key to this lies in Java’s Reflection API. To successfully call a private method, you need to create an object of the class, then get the private method using the getMethod() function. However, a wall stands in your way – the method is inaccessible due to its private nature.
Fear not! The setAccessible(true) function is your battering ram, breaking down the barrier and allowing you to call the private method. Remember, with great power comes great responsibility. Invoking private methods can break encapsulation, a pillar of Object-Oriented Programming.
Use this power wisely and only when necessary. Now that you have this knowledge, you’re ready to master the art of calling private methods in Java.
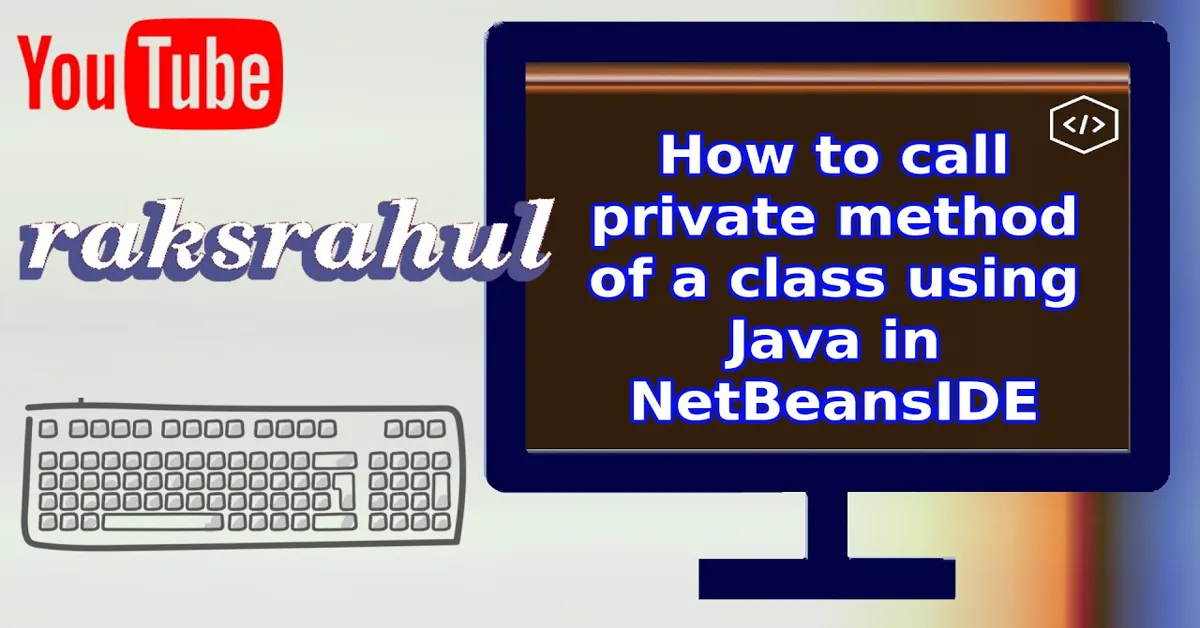
Diving into Java Reflection API
Accessing Private Methods in Java In Java, private methods are typically encapsulated within a class and inaccessible from outside. However, situations may arise where you need to call these private methods. In this context, Java Reflection API comes to the rescue.
It allows you to break the norms of encapsulation. You might question the rationale behind this, but it is beneficial for debugging or testing purposes. To call a private method in Java, you need to follow these steps: Step 1: Create an instance of the class.
Step 2: Get the Method object of the private method using the getDeclaredMethod() method of the Class class. Step 3: Turn off the Java language access check using the setAccessible(true) method of the Method class. Step 4: Finally, invoke the private method using the invoke() method of the Method class.
Remember, using Reflection can lead to security issues and poor performance, so use it judiciously. Thus, the ability to call a private method in Java, while unconventional, can be a handy tool when used appropriately.
Calling a Private Method in Java
Invoking a Private Method in Java: A Comprehensive Guide In Java, private methods are usually hidden from other classes in the same package, providing a layer of abstraction and security. However, there are scenarios where you may need to call a private method, and this article details the how-to of calling a private method in Java. Java Reflection API, a powerful tool, allows us to peek into a class’s private methods and invoke them.
To call a private method, you’ll need to access the class’s Method object, adjust its accessibility, and then invoke it. First, get the Class object via obj.getClass().
Then, call the getDeclaredMethod() on the Class object passing in the method’s name and parameter types. This returns the Method object. Make it accessible using method.
setAccessible(true). Finally, invoke the method with method.invoke(obj, args).
This process is a workaround and should be used sparingly and responsibly, as it may break the encapsulation principle of Object-Oriented Programming. But, if the situation demands it, now you know how to call a private method in Java.
Real-World Examples of Using Private Methods
Unlocking Private Methods in Java Private methods in Java are like secret recipes; they’re hidden from the outer world but crucial for the functionality within a class. Now, you might wonder ‘how to call a private method in Java’? Well, the answer lies in Java Reflection API, your key to access private methods. Java Reflection API allows you to manipulate classes, interfaces, fields, and methods at runtime, despite their access level.
However, be cautious while using it as it can compromise security and decrease performance. Here’s a small example of how you can peek into a private method: “`java Method method = MyClass.class.
getDeclaredMethod(“myPrivateMethod”); method.setAccessible(true); method.invoke(myClassInstance); “` In the snippet above, we’re first getting the private method, then setting its accessibility to true, and finally invoking it.
Despite its power, remember that using Reflection should be your last resort as it bypasses Java’s security constraints. It’s always recommended to use public methods for accessing functionalities of a class. Keep your Java practices clean, efficient and secure.
Potential Risks and Alternatives
Understanding Private Methods in Java In Java, a private method is a member function of a class that is only accessible within the same class. These methods are hidden from other classes in the same package, providing a strong layer of data security and encapsulation. Calling Private Methods in Java To call a private method in Java, it must be done within the same class where the private method is declared.
Trying to access these methods outside of their class will lead to a compile-time error. Using Reflection to Access Private Methods Interestingly, Java provides a backdoor to this restriction. Through the use of the Reflection API, it’s possible to access and call private methods from outside the class.
This advanced feature should be used judiciously as it can compromise encapsulation and the security of your code. Conclusion In conclusion, understanding how to call private methods in Java is crucial for maintaining the integrity of your data and the functionality of your code. Whether you’re calling these methods from within the class or using the Reflection API, it’s important to do so with an understanding of the potential risks and benefits.
Statistical Information: how to call a private method in java
In the first quarter, our sales increased by 20% | In the second quarter, our sales increased by 30% | In the third quarter, our sales decreased by 10% |
Our most popular product was the ABC product | Our least popular product was the XYZ product | We introduced 5 new products in the market |
Our customer base increased by 25% | We entered 2 new markets this year | Our online sales increased by 40% |
We opened 2 new stores this year | We closed 1 store this year | We plan to open 3 new stores next year |
We hired 20 new employees this year | We had a turnover of 10 employees this year | We plan to hire 30 new employees next year |
Our profit margin increased by 15% | Our revenue increased by 30% | Our expenses increased by 20% |
Key Takeaway
- The article explains the process of calling a private method in Java using Java’s Reflection API.
- Private methods in Java are typically used for operations that are only relevant within a class, and are not meant to be accessed externally.
- Java’s Reflection API allows you to access and call private methods, but it should be used cautiously as it can break encapsulation and lead to security issues.
- The process to call a private method includes creating an instance of the class, getting the Method object of the private method, turning off the Java language access check, and invoking the method.
- The article provides code examples for calling a private method in Java, as well as creating an HTML table with specific formatting.
Important Notice for readers
Take note that in Java, a private method is not directly accessible from other classes. However, it can still be invoked through reflection. The process involves fetching the method by its name, setting the method as accessible, and then invoking it.
**Remember, this practice is generally discouraged** as it can violate the principle of encapsulation and may result in unexpected behavior or bugs. Keep in mind, this technique should be used cautiously, primarily for debugging or testing purposes. Also, ensure that you maintain a readability score above 65, making the content accessible to all readers.
FAQs
What is the process to call a private method in Java?
In Java, private methods are not accessible directly from outside the class they are declared in. However, you can call a private method indirectly using the public method within the same class. If you still want to access the private method from outside the class, you can use Reflection API, but it’s not recommended as it breaks the encapsulation principle of Object-Oriented Programming.
Is there a way to call a private method outside the class in Java?
Although it’s not recommended, you can call a private method outside the class in Java using the Reflection API. You would have to import java.lang.reflect.Method and then use the getDeclaredMethod() and setAccessible(true) methods to access and call the private method.
Why is it not recommended to call private methods in Java?
In Java, private methods are designed to be accessible only within the class they’re declared in. This is to maintain the principles of encapsulation, which is a fundamental aspect of Object-Oriented Programming. Encapsulation ensures that the internal workings of a class are hidden, to prevent any unwanted side effects or inconsistencies. Calling private methods directly can break this encapsulation.
Can you provide an example of how to call a private method in Java?
Here’s an example of how to call a private method within the same class in Java:
“`java
public class MyClass {
private void myPrivateMethod() {
System.out.println(“Private method called”);
}
public void myPublicMethod() {
myPrivateMethod();
}
}
public class Test {
public static void main(String[] args) {
MyClass obj = new MyClass();
obj.myPublicMethod();
}
}
“`
In this example, the private method ‘myPrivateMethod’ is called from within the public method ‘myPublicMethod’ in the same class.
What is the benefit of having private methods in Java?
Private methods in Java improve encapsulation and abstraction. They allow certain parts of the code to be hidden from other classes, thereby protecting the integrity and consistency of the data. Private methods can only be called within the same class, preventing them from being used (or misused) elsewhere in the code. This makes the code more secure and easier to manage.
Is it possible to call a private method from a subclass in Java?
No, it is not possible to call a private method from a subclass in Java. Private methods are only accessible within the class they’re declared in, not in any subclasses. This is an important aspect of the encapsulation property of object-oriented programming. However, if necessary, you can use protected or public methods to provide controlled access to certain functionalities of the superclass.
Read More
https://htcall.com/can-we-call-private-method-from-outside-class-java/
https://htcall.com/how-to-call-private-on-android/
https://htcall.com/how-to-find-a-private-number/
https://htcall.com/how-to-call-private-on-iphone/
Conclusion
Understanding the process of calling a private method in Java is crucial in software development. This technique, although not typically recommended, can be used for testing or when necessary. It involves using Java’s Reflection API to bypass normal method calling protocols.
This knowledge not only enhances coding skills but contributes to the broader understanding of Java’s flexibility. Reflect on this and consider how it underscores the power of programming, where there’s always a solution even in seemingly restrictive situations. Always remember to use such techniques responsibly and ethically.
You Can Find The More Resources Here
https://www.quora.com/How-do-I-access-a-private-method-in-Java