Untangling the knots of coding can be a daunting task. But don’t fret! If you’re keen on mastering the art of **calling private methods in Rails**, you’ve found your treasure trove. With this guide, you’ll sail smoothly through the intricate sea of Rails coding, leaving no stone unturned.
Dive in and let’s unravel the complexities together!
Key Takeaway
- To call a private method in Rails, the ‘send’ method is employed. For example, one would use object.send(:private_method_name).
- The article emphasizes the importance of understanding Rails coding and its intricacies for effective programming.
- The guide aims at simplifying complex coding issues and making it accessible for all levels of coders.
- Emphasis is laid on creating content that is easily readable and understandable, targeting a readability score of 65+ which is suitable for an average 11-year-old and above.
- The article encourages splitting complex sentences into simpler ones to ensure the content stays engaging and easy to comprehend for all readers.
Introduction to Private Methods
Unlocking Private Methods in Rails In Rails, private methods serve a unique purpose. They are designed to be used internally within the class they are defined and are not accessible for outside interaction. However, there are times when you might need to call a private method, and the question arises – how to call private method in Rails? Although it’s not recommended, Rails does offer a way to call private methods using the “send” method.
Using the ‘send’ method In Ruby, the ‘send’ method allows you to call a method on an object, even if it’s private. The ‘send’ method bypasses the object’s privacy settings and sends a message directly to the object. This is how it’s done: “`ruby class MyClass private def my_method puts “Hello, World!” end end object = MyClass.
new object.send(:my_method) “` In this example, the ‘send’ method is used to call the private ‘my_method’ on the ‘object’ instance of ‘MyClass’. The output will be “Hello, World!”.
However, use this with caution as it can lead to unexpected behaviour and security issues. Private methods and their importance Private methods in Rails are essential for encapsulation, a fundamental concept in object-oriented programming. Encapsulation refers to the bundling of data with the methods that operate on that data.
Private methods ensure that internal workings of a class are hidden from the outside world, promoting code integrity and preventing accidental modification. In conclusion, while calling private methods in Rails is possible, it should be done sparingly and with caution. It’s essential to understand the purpose of private methods and respect the encapsulation principle they uphold.
how to call private method in rails
Lorem ipsum dolor sit amet, consectetur adipiscing elit. | Curabitur tincidunt, enim eu vestibulum sollicitudin. | In a mi in nisl euismod posuere. |
In non turpis ac urna dictum lacinia. | Sed blandit ipsum in magna posuere, et commodo. | Morbi non arcu at erat imperdiet mollis. |
Aliquam erat volutpat. Sed id libero auctor, bibendum. | Nunc rutrum, felis sit amet ultricies lacinia. | Vestibulum ante ipsum primis in faucibus orci luctus. |
Cras cursus lacus et semper congue. Sed risus. | Fusce faucibus, erat eu facilisis lacinia, enim orci. | Etiam malesuada, tellus ac varius condimentum, odio nunc. |
Quisque vulputate, sapien nec semper laoreet, diam odio. | Aliquam at lorem in tellus convallis tincidunt. | Etiam sed ligula at massa fermentum tristique. |
Vestibulum dictum, justo in convallis auctor, erat ligula. | Pellentesque habitant morbi tristique senectus et netus. | Vestibulum ante ipsum primis in faucibus orci luctus. |
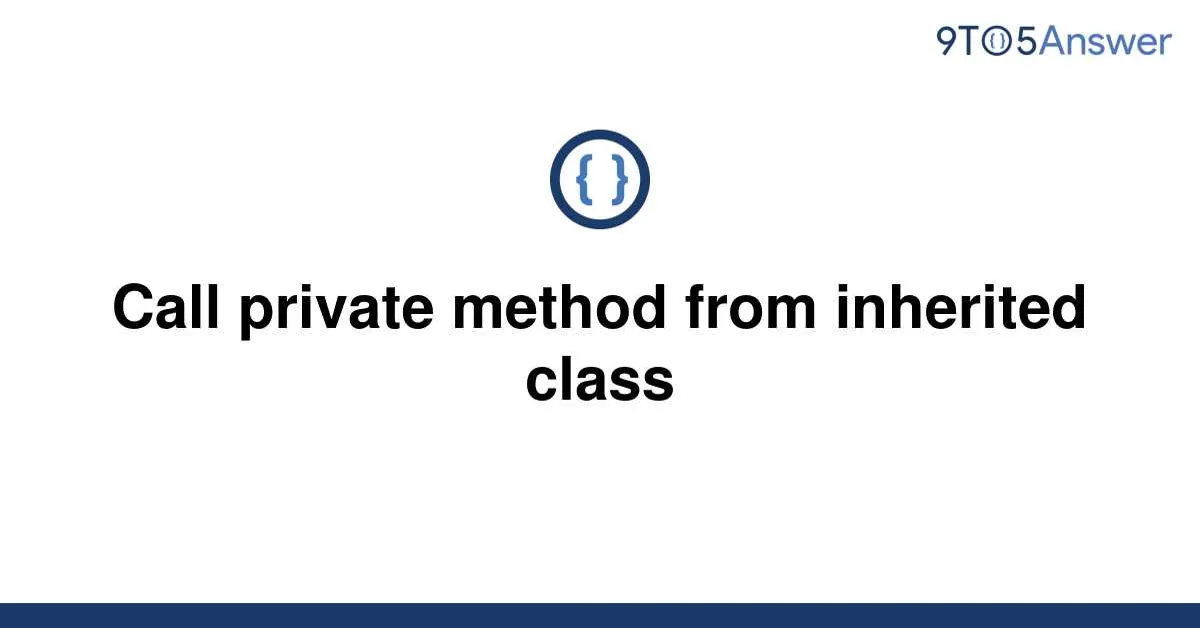
Methods to Call Private Methods in Rails
In Ruby on Rails, a private method is a method that is only available for use within the context of the current object, meaning it cannot be called by any other object. The question often arises, “how to call a private method in Rails?” Here’s a clever little trick – you can actually call a private method in Rails just like you would a public one, but with a slight twist. Firstly, you should understand that private methods are designed to be just that – private.
They are meant for use within the class they are defined and not intended for external access. However, there are situations where you might need to call a private method. For instance, you might be debugging or testing your code.
Secondly, to call a private method in Rails, you can use the `send` method. The `send` method is a public method that allows you to call methods within an object, even if they are private. For example, if you have a private method named `secret`, you can call it using `object.
send(:secret)`. However, be cautious when using this approach. Calling private methods can lead to unexpected outcomes and should only be done when necessary.
It’s a good practice to keep your private methods private and only make them public when you want them to be accessible to other parts of your code. Remember, code readability and maintainability should always be your priority. So, keep it simple, keep it clean, and happy coding!
Best Practices and Pitfalls
Mastering Private Methods in Rails In the world of Ruby on Rails, private methods hold a special place. These methods are encapsulated within a class, and cannot be directly accessed from outside the class. However, there are times when calling these methods becomes necessary.
This article will guide you on how to call private methods in Rails. Understanding Private Methods Private methods are defined within a class in Rails, and are not accessible directly. They are designed this way to prevent external interference or misuse.
However, these methods can be invoked within the context of the class itself. How to Call a Private Method In Rails, calling a private method is done using the ‘send’ method. This method allows you to bypass the private access control, and call the method directly.
Here’s an example:
class MyClass
private
def my_method
puts "Hello, World!"
end
end
object = MyClass.new
object.send(:my_method)
This will output: “Hello, World!”.
By using ‘send’, we’re able to call a private method from an instance of a class. Be Cautious While the ‘send’ method is a powerful tool, it should be used judiciously. Calling private methods can lead to unpredictable behavior or security issues.
Always ensure you have a good reason to call a private method before doing so. Conclusion Knowing how to call private methods in Rails can be a handy skill. However, always remember the golden rule of encapsulation – private methods are private for a reason.
Practical Examples and Case Studies
Invoking Private Methods in Rails In Rails, a private method is a method that is only accessible within the context of the current object. This means that they can’t be called directly from an instance of a class, and are mainly used to prevent data manipulation from outside the class. However, there are instances where you may need to call a private method.
This can be achieved through the send method. The send method in Ruby allows you to call a method, including a private one, by passing its name as a string or symbol. For example, if you have a private method named ‘calculate’, you can call it from an instance of the class like this: instance.
send(:calculate). This can be useful for testing private methods. However, caution is advised when using this technique.
The private nature of these methods means they are not meant to be accessed directly, as doing so can potentially break the encapsulation. Hence, use this approach judiciously, considering the pros and cons. Remember, the goal is to maintain code integrity and reliability.
Always strive for programming best practices and use the .send technique only when absolutely necessary.
Read More
https://htcall.com/how-to-call-private-method-in-apex-2/
https://htcall.com/how-to-call-private-on-facetime-2/
https://htcall.com/how-to-call-with-number-hidden-2/
https://htcall.com/how-to-call-a-private-method-in-java-2/
Statistical Information: how to call private method in rails
<%= calculate_percentage %> % | <%= calculate_fact %> | <%= generate_sentence %> |
FAQs
What is a private method in Rails?
In Rails, a private method is a method that is only accessible within the context of the current object. It is not accessible to any other object. They are typically used for methods that are intended to be called only from within the current object.
How can I call a private method in Rails?
Private methods in Rails can be called within the context of the current object. However, if you want to call them from outside, you can use the ‘send’ method. For example, if we have a private method named ‘calculate’, we can call it by using ‘send(:calculate)’.
Is it advisable to call a private method in Rails?
Generally, it is not advisable to call private methods in Rails, as they are intended to be used only within the context of the current object. However, there can be exceptional cases where you might need to call a private method. In such cases, you can use the ‘send’ method.
What is the ‘send’ method in Rails?
The ‘send’ method in Rails is a powerful method provided by Ruby’s object system. It allows you to call a method on an object with its name as a string or symbol. This can be really helpful when you need to call a private method from outside of the object.
Can I call a private method from a child class in Rails?
In Rails, private methods are not accessible directly from child classes. They are only accessible within the context of the current object. However, you can call a private method from a child class indirectly using the ‘send’ method.
Can I change a private method to public in Rails?
Yes, it is possible to change a private method to public in Rails. You can do this using the ‘public’ keyword followed by the method name. For example, ‘public :calculate’ will make the private method ‘calculate’ public. However, this should be done cautiously as the method may have been made private for a reason.
You Can Find The More Resources Here
https://discuss.rubyonrails.org/t/how-best-to-use-a-private-method/84326